TLDR
In short, Plain Flags helps you turn features on and off in production.
You can install Plain Flags as a set of three services in your production environment, conveniently available as Docker containers.
In the Plain Flags dashboard, which is a web application, you can create feature flags, turn them on and off, and, if you wish, restrict them to only some subsets of your users.
In your software's source code, you will need to surround your feature code with some simple condition, to allow toggling it.
Plain Flags Parts
Plain Flags is made of a few parts with which you may want to get acquainted before using it.
The parts you install on your own back end are three services: flag-management, flag-states, and flags-dashboard, as named in the Docker Compose file shown in the next section.
There's also the part that you include in your own software.
If your application's development technology is Node.JS, you can install this part from the npm repository and use it as a library.
If Plain Flags doesn't provide an SDK for your technology, you can get the feature flag state easily from the backend with a simple HTTP GET request.
Plain Flags aims to grow its collection of SDK's to support more programming languages and frameworks in future versions.
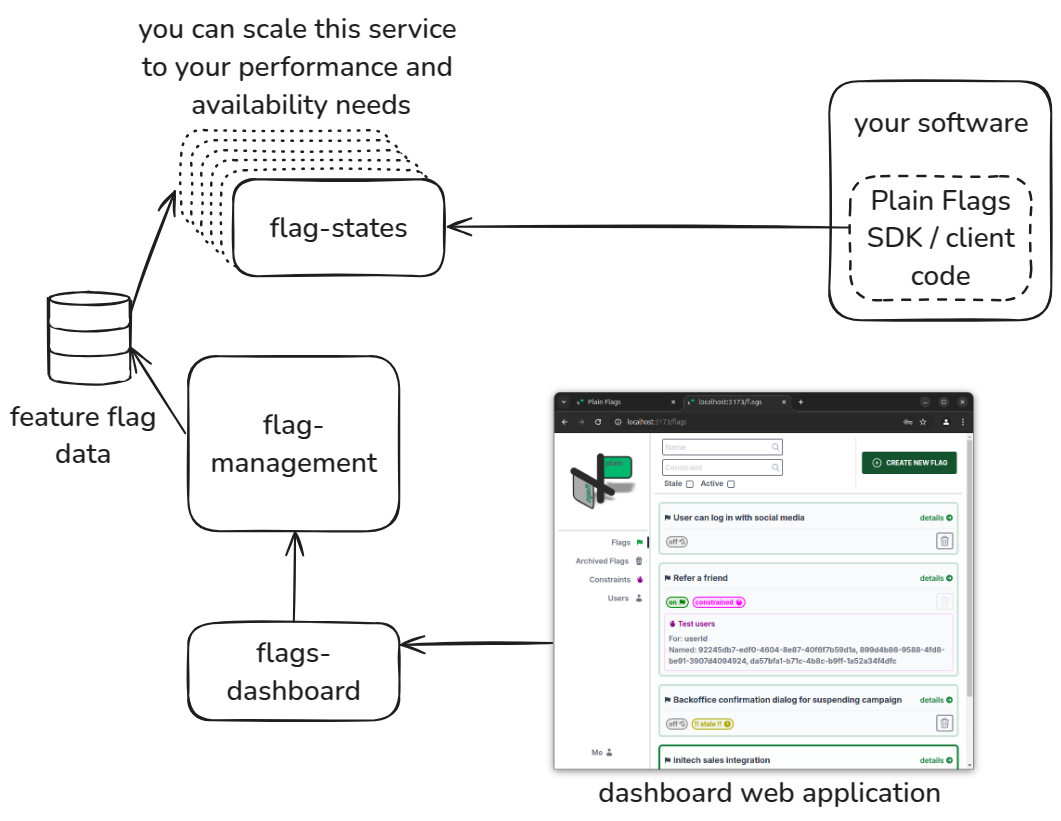
Data Storage
By default, Plain Flags uses SQLite database technology to store your feature flag data.
This choice of database allows for less complexity in terms of running services, since Plain Flags is designed to be self hosted.
SQLite stores the data in a file, and Plain Flags works with this file inside a folder. Set the environment variable DATA_FOLDER_PATH if you want to override the default data folder path.
The example Docker Compose file in the next section shows a way to map the data folder as a volume.
Plain Flags also supports storing your feature flag data in a PostgreSQL database. Below is an example of a docker compose yaml file that allows Postgres data storage.
Set Up And Run
You can run each component service as a Docker container.
You can also run each component as a Node.JS application on whichever environment is convenient to you by building them from the freely available source code on Github.
Configuration
The environment variables that configure your instance are evident in the example Docker Compose file.
Flag management service:
SERVICE_PORT
The port where flag management exposes its REST API for creating, changing feature flags. Default 5000
STALE_FLAG_DAYS
Number of days after which a feature flag may be considered stale. Stale flags are those that haven't changed value for a long time, and the dashboard warns users about forgotten feature flags, so they can keep their source code uncluttered.
SUPERADMIN_EMAIL
The email address of the superadmin user.
SUPERADMIN_PASSWORD
The initial password of the superadmin user.
All users are advised to change their password after their first login
SUPERADMIN_PASSWORD_FILE
Path to a file containing the initial password of the superadmin user. If set, it overrides SUPERADMIN_PASSWORD
DEFAULT_USER_PASSWORD
The initial password for users created by admin.
All users are advised to change their password after their first login
DEFAULT_USER_PASSWORD_FILE
Path to a file containing the initial password for users created by admin. If set, it overrides DEFAULT_USER_PASSWORD
DISABLE_USER_REGISTRATION
Set to true to disable user's ability to register in the dashboard. With this setting, only admins can create users in the Users section.
JWT_SIGNING_SECRET
JSON Web Token signing secret for user authentication.
This is a secret. It is recommended to store and pass it using a secret storage mechanism
JWT_SIGNING_SECRET_FILE
Path to a file containing the JSON Web Token signing secret for user authentication.
If set, it overrides JWT_SIGNING_SECRET
APIKEY
API key. For this service, only the test application uses this variable, so it's only relevant for development.
This is a secret. It is recommended to store and pass it using a secret storage mechanism
APIKEY_FILE
Path to a file containing the API key. For this service, only the test application uses this variable, so it's only relevant for development.
If set, it overrides APIKEY
The following variables are only required if you choose to store your feature flag data in a PostgreSQL database
DATABASE_TYPE
If you prefer to store your feature flag data in a PostgreSQL database, set the value to pg.
For the default SQLite option, leave this variable undefined.
DATABASE_HOST
Base URL of your database server.
DATABASE_PORT
The port on which your database server is available.
DATABASE_NAME
Name of your database.
DATABASE_USER
User name for the database.
DATABASE_PASSWORD
Password to your database.
This is a secret. It is recommended to store and pass it using a secret storage mechanism
DATABASE_PASSWORD_FILE
Path to a file containing the password to your database.
If set, it overrides DATABASE_PASSWORD
DATABASE_POOL_SIZE
Connection pool size for this service's database.
Make sure this value across all service instances does not exceed your database's configured max connections value.
Flag states service:
SERVICE_PORT
The port where flag states exposes its REST API for obtaining feature flag state information. Default 5001
APIKEY
Shared API key between this service and your software. Requests for feature flag states are authenticated with this API key. The SDK takes value this as an argument on initialization.
This is a secret. It is recommended to store and pass it using a secret storage mechanism
APIKEY_FILE
Path to a file containing the shared API key between this service and your software.
If set, it overrides APIKEY
CACHE_TTL
Time to live value in milliseconds for the service's feature flag state cache.
A value of zero ensures immediate update after an operator changes a flag value, but overburdens the database.
A greater value (e.g. 1000) ensures the ability to sustain more client connections. Set a positive value if you have many applications that use the feature flags.
The following variables are only required if you choose to store your feature flag data in a PostgreSQL database
DATABASE_TYPE
Must be the same value as for the Flag Management Service
DATABASE_HOST
Must be the same value as for the Flag Management Service
DATABASE_PORT
Must be the same value as for the Flag Management Service
DATABASE_NAME
Must be the same value as for the Flag Management Service
DATABASE_USER
Must be the same value as for the Flag Management Service
DATABASE_PASSWORD
Must be the same value as for the Flag Management Service
DATABASE_PASSWORD_FILE
Must be the same value as for the Flag Management Service
DATABASE_POOL_SIZE
Connection pool size for this service's database.
Make sure this value across all service instances does not exceed your database's configured max connections value.
Dashboard service:
VITE_API_URL
URL to the API endpoint of the flag management service
VITE_DISABLE_USER_REGISTRATION
When set to true, the front end does not display the user registration UI
In Your Software
Node.JS (TypeScript)
If your software is using Node.JS as a development technology, you can install the Plain Flags SDK from the npm registry.
npm install plain-flags-node-sdk
Construct a PlainFlags object. In the constructor call, you choose an update policy for your feature flag states, so your application can be aware of which features you want enabled or disabled.
Initialize the PlainFlags object. If you opt for manual update policy, you can also call its updateState method at any point you choose.
You can study the behaviour tests in the freely available source code and see exactly how calls to Plain Flags SDK are made.
Go (Golang)
If your software is written in Go, you can install the Plain Flags SDK using this command:
go get github.com/andreileonte1981/plain-flags/sdk/go
Use the SDK's factory function NewPlainFlags to create a PlainFlags struct.
PlainFlags polls the flag state service at a specified interval to download the latest feature flag state.
To forego polling and only update manually, configure the PlainFlags struct with a 0 PollInterval value at its creation.
Initialize the PlainFlags to start using the feature flag state. If you opt for manual updates, you can also call the UpdateState receiver function at any point you choose.
PlainFlags functions can be called as goroutines, and errors are available in the channels passed as arguments.
You can study the behaviour tests in the freely available source code and see exactly how calls to Plain Flags SDK are made.
Python
If your software is written in Python, you can install the Plain Flags SDK using this command:
pip install plain-flags-sdk
Use the PlainFlags constructor to create a PlainFlags object.
PlainFlags polls the flag state service at a specified interval to download the latest feature flag state.
To forego polling and only update manually, configure the PlainFlags object with a 0 poll_interval value at its creation, in the PlainFlagsConfig object you pass.
Initialize the PlainFlags to start using the feature flag state. If you opt for manual updates, you can also call the UpdateState receiver function at any point you choose.
You can study the behaviour tests in the freely available source code and see exactly how calls to Plain Flags SDK are made.
Other Platforms
If your software is written in another programming language and Plain Flags does not yet offer an SDK library for it, you can still update the feature flag state in your application with a GET request to the flag state service endpoint "/api/sdk".
The GET request to the flag states service must be authenticated by including the x-api-key header and set its value to the same API key you configure on the back end.
You can read and imitate the Node.JS SDK source code in the Plain Flags repository.
It is up to you and your developers to write the conditions under which your feature code is executed. In most cases, a simple if statement encompassing that feature code is effective. Plain Flags only provides Boolean type flags, in accordance with its commitment to simplicity.
Controlling your features
Navigate to the dashboard web app and log in.
Create a new feature flag.
Choose the name carefully: this name will be unique over the lifetime of your Plain Flags installation.
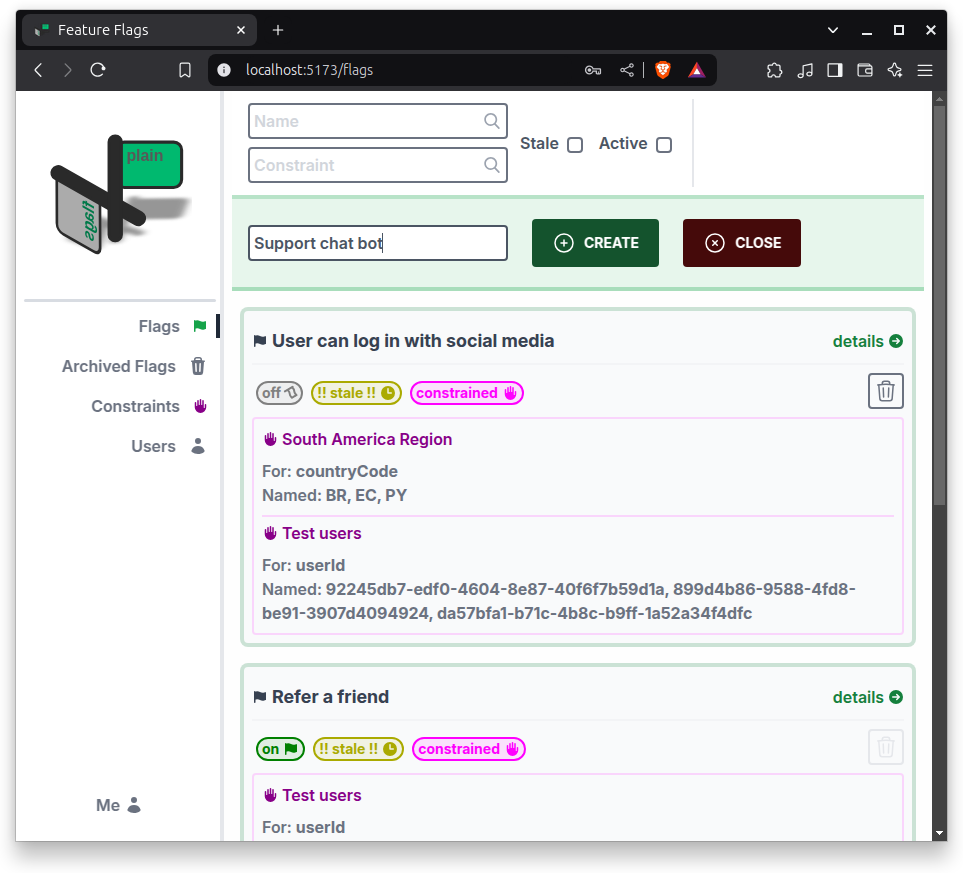
You can, if you prefer, compose the name of multiple sections such as purpose, team ID, feature description and so on. Plain Flags is agnostic with respect to any details of your organization.
Once created, you must modify your source code so it only exhibits that feature when the feature flag is on.
Before turning on the feature flag from the details section, you can constrain it to subsets of your users.
Useful examples of which users you can constrain a feature to include:
Internal users, for testing the feature in production
Anyone using your software just in a specified set of regions or countries where you serve particular markets
Users in an experimental sample for A/B tests
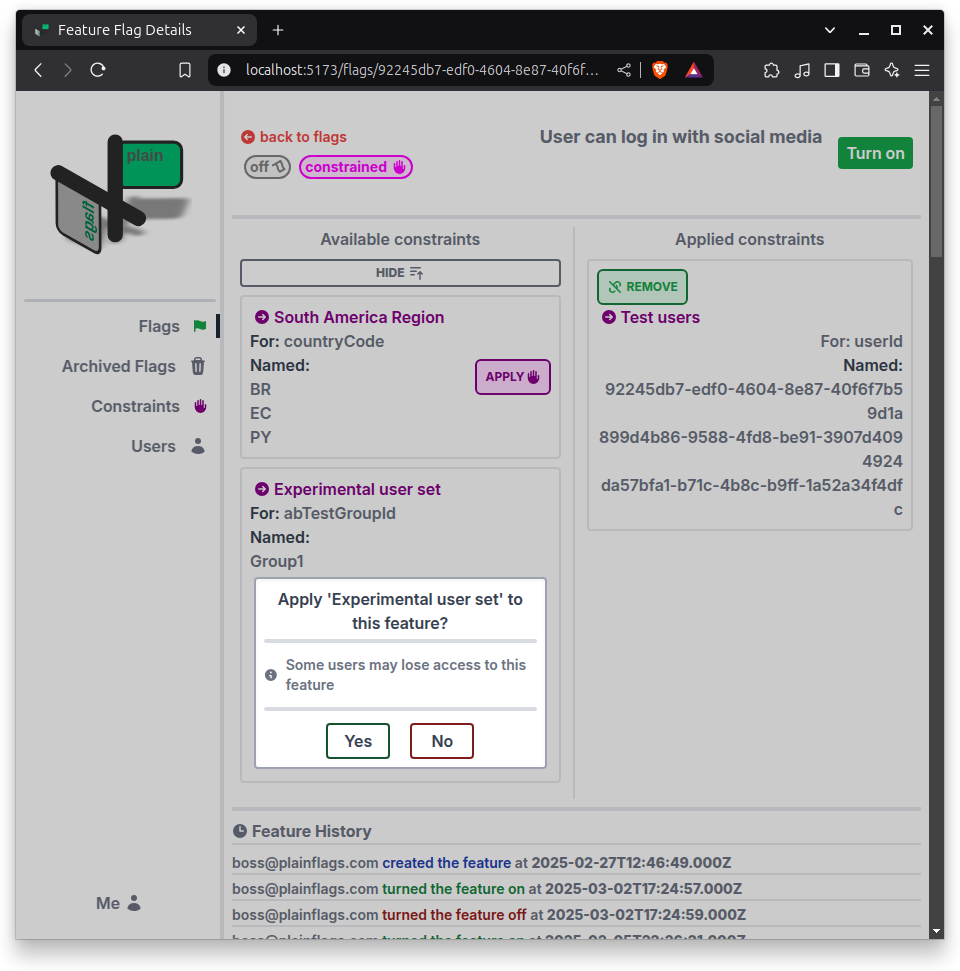
Create one or more constraints in the Constraints section of the dashboard, then apply those constraints to your feature flag in its details page.
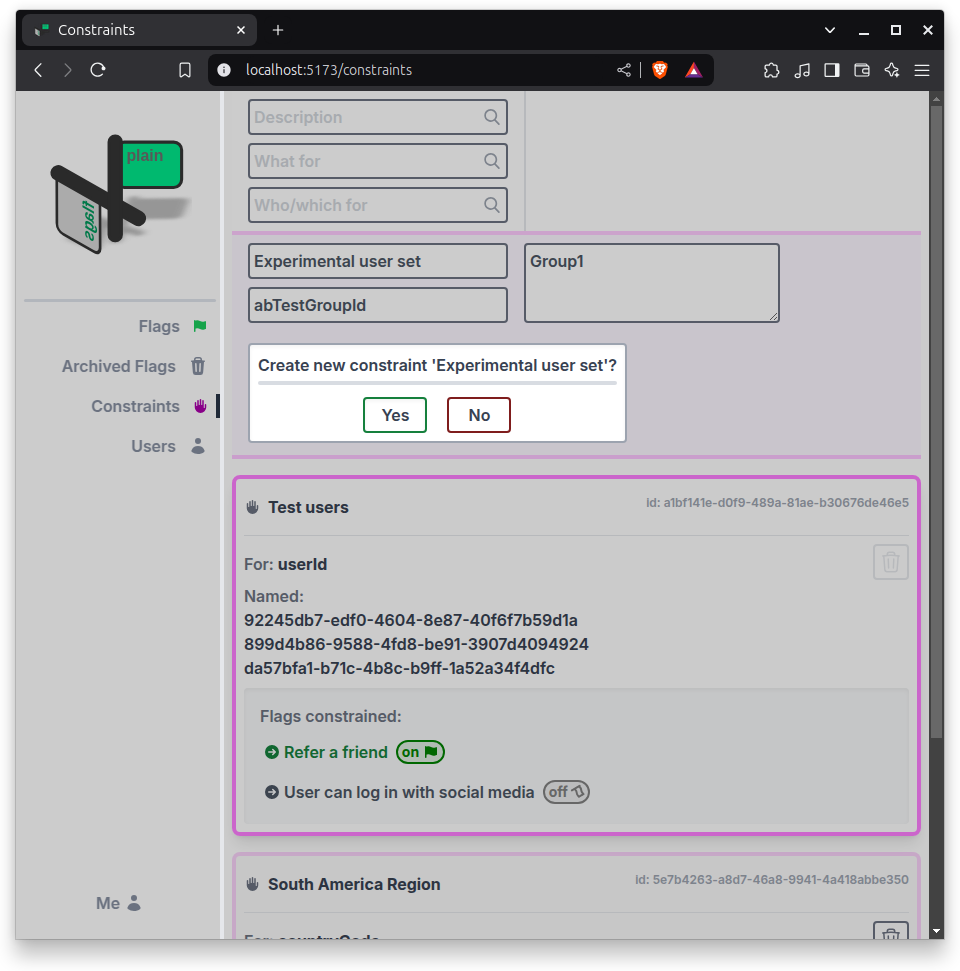
Below is an example of how to use constrained flags in your software:
Workflow
This section discusses feature flags in general, in contrast with the traditional software release methodology.
Deploy vs Release
Software teams traditionally release their new features when they deploy some new version of their application.
We are likely to miss the difference the two concepts: release and deployment. In fact, this was inevitable at the time of physical releases on cd-rom or floppy discs.
But, even when done in one step, they refer to two concepts from two different perspectives.
Operations team makes a new binary version available publicly on the Internet
Deployment is a concrete technical concept
The developers make the new features available to their customers
Release is an abstract human term
With feature flags and ubiquitous Internet, a development team doesn't have to release all new features at the time the software is deployed.
Traditional Workflow
Below is an example of a common release cycle, excluding some details for brevity:
Developers write a number of new features in a couple of weeks.
A release candidate is deployed to the staging environment. Often, the pre-release source control branch is frozen (no new merges except fixes) until deployment.
The QA team tests the release candidate, going through the included new features. They often find a few bugs.
Devs now have a couple of days to fix the bugs.
If the issues aren't fixed on time, the affected features have to be rolled back or deactivated, and will wait for the next release in two more weeks.
At this point, there is no guarantee that the release candidate is bug free - the fixes may as well have introduced unexpected new bugs.
Team leadership must choose between delaying the deployment and rushing insufficiently tested software.
Here are a few problems with the traditional release model that feature flags can solve:
- Testing and bug fixing peaks before a scheduled deployment. This is often done under high pressure, leading to low software quality and team burnout. The longer the time between deployments, the more new stuff your team has to test and fix in a hurry. With feature flags, you can deploy a hundred new features with a new version, but release each of them at any time you want, allowing flexibility for your team's testing and bug fixing capacity.
- Testing and bug fixing is made in an environment different from the production one. Such staging environments are costly. Even when accepting the extra cost, tests are not made under exact production conditions, leading to unpleasant surprises after releasing.
- Rolling back is so cumbersome for a large batch release that most times a bug is allowed to continue in production until a hot fix is written, under great pressure. In many cases the hot fix introduces other bugs, on top of extra technical debt inherent in most rushed software development. With feature flags, you just turn the new feature off and fix it with less pressure.
Feature Flags
Feature flags are only a small tool in the greater set of newer software methodologies, such as continuous delivery, and may not fit ideally in the traditional sprint based or heavily planned ahead models.
To make the most of feature flags, a software team can consider some changes in its own workflow.
- Trust and empower your team members to take more responsibility for the features they make. Let them deploy their creations in production and safely test them behind feature flags' user constraints. The ability of feature flags to turn capabilities on and off quickly means much lower risk of bugs manifesting and doing damage.
- Encourage more focused collaboration with QA and operations experts. Feature flags' granular control benefits more from small teams of mixed specialists that tackle one feature at a time.
- Modular software design comes naturally with feature flags. Since developers need to put all code related to a feature behind conditions, they will get accustomed to thinking about their changes in a modular, decoupled fashion, which brings extra benefits, such as easier maintenance and testability, increasing the overall quality of your products.